auto = null;
timer = null;
var focus = new Function();
focus.prototype = {
init : function () {
this.aTime = this.aTime || 10;
this.sTime = this.sTime || 5000;
this.oImg = document.getElementById('focus_m').getElementsByTagName("ul")[0];
this.oImgLi = this.oImg.getElementsByTagName("li");
this.oL = document.getElementById('focus_l');
this.oR = document.getElementById('focus_r');
this.createTextDom();
this.target = 0;
this.autoMove();
this.oAction();
},
createTextDom : function () {
var that = this;
this.oText = document.createElement("div");
this.oText.className = "focus_s";
var ul = document.createElement('ul');
var frag = document.createDocumentFragment();
for (var i = 0; i < this.oImgLi.length; i++) {
var li = document.createElement("li");
li.innerHTML = '<b></b>';
if (i == 0) {
li.className = "active";
};
frag.appendChild(li);
};
ul.appendChild(frag);
this.oText.appendChild(ul);
this.o.insertBefore(this.oText, this.o.firstChild);
this.oTextLi = this.oText.getElementsByTagName("li");
},
autoMove : function () {
var that = this;
auto = setInterval(function () {
that.goNext()
}, that.sTime);
},
goNext : function () {
this.target = this.nowIndex();
this.target == this.oTextLi.length - 1 ? this.target = 0 : this.target++;
this.aStep = (this.target - this.nowIndex()) * this.step;
this.removeClassName();
this.oTextLi[this.target].className = "active";
this.startMove();
},
goPrev : function () {
this.target = this.nowIndex();
this.target == 0 ? this.target = this.oTextLi.length - 1 : this.target--;
this.aStep = (this.target - this.nowIndex()) * this.step;
this.removeClassName();
this.oTextLi[this.target].className = "active";
this.startMove();
},
startMove : function () {
var that = this;
var t = 0;
this.timer = '';
function set() {
if (t > 100) {
clearInterval(that.timer);
} else {
for (var i = 0; i < that.oImgLi.length; i++) {
that.oImgLi[i].style.display = 'none';
};
that.oImgLi[that.target].style.display = 'block';
that.setOpacity(that.oImg, t);
t += 5;
};
};
timer = setInterval(set, that.aTime);
},
setOpacity : function (elem, level) {
if (elem.filters) {
elem.style.filter = 'alpha(opacity=' + level + ')';
elem.style.zoom = 1;
} else {
elem.style.opacity = level / 100;
};
},
nowIndex : function () {
for (var i = 0; i < this.oTextLi.length; i++) {
if (this.oTextLi[i].className == 'active') {
return i;
break;
}
};
},
oAction : function () {
var that = this;
for (var i = 0; i < this.oTextLi.length; i++) {
this.oTextLi[i].index = i;
this.oTextLi[i].onclick = function () {
clearInterval(auto);
clearInterval(timer);
that.setOpacity(that.oImg, 100);
that.target = this.index;
that.removeClassName();
this.className = 'active';
that.startMove();
}
};
mouseEnter(that.o, 'mouseleave', function (e) {
clearInterval(auto);
that.autoMove();
});
this.oL.onclick = function () {
that.goPrev();
};
this.oR.onclick = function () {
that.goNext();
};
},
removeClassName : function () {
for (var i = 0; i < this.oTextLi.length; i++) {
this.oTextLi[i].className = ""
};
}
};
var focusRun = new focus();
focusRun.o = document.getElementById("focus");
function mouseEnter(ele, type, func) {
if (window.document.all)
ele.attachEvent('on' + type, func);
else {
if (type === 'mouseenter')
ele.addEventListener('mouseover', this.withoutChildFunction(func), false);
else if (type === 'mouseleave')
ele.addEventListener('mouseout', this.withoutChildFunction(func), false);
else
ele.addEventListener(type, func, false);
};
};
function withoutChildFunction(func) {
return function (e) {
var parent = e.relatedTarget;
while (parent != this && parent) {
try {
parent = parent.parentNode;
} catch (e) {
break;
}
}
if (parent != this)
func(e);
};
};
var login = function () {
var loading = new tui.Loading();
var m = document.getElementById('login_main')
var s = document.getElementById('login_sub');
var li = m.getElementsByTagName('li');
var a = m.getElementsByTagName('a');
var cnzz = document.getElementById('login_cnzz');
var quan = document.getElementById('login_quan');
var input = s.getElementsByTagName('input');
loading.init(s);
li[0].onclick = function () {
cnzz.style.display = 'block';
quan.style.display = 'none';
a[1].className = '';
a[0].className = 'active';
o['cnzz']['e'].innerHTML = '';
o['quan']['e'].innerHTML = '';
};
li[1].onclick = function () {
cnzz.style.display = 'none';
quan.style.display = 'block';
a[1].className = 'active';
a[0].className = '';
o['cnzz']['e'].innerHTML = '';
o['quan']['e'].innerHTML = '';
};
for (var i = 0; i < input.length; i++) {
if (input[i].type == 'checkbox') {
continue
};
if (!checkPlaceHolderExist() && input[i].value == '') {
input[i].value = input[i].getAttribute('placeholder');
};
input[i].onfocus = function () {
var self = this;
document.onkeydown = function (e) {
e = e || event;
if (e.keyCode == 13) {
if (a[0].className == 'active') {
cnzzSubmit();
} else {
quanSubmit();
}
}
};
if (!checkPlaceHolderExist() && (this.value == this.getAttribute('placeholder'))) {
this.value = '';
};
};
input[i].onblur = function () {
document.onkeydown = null;
if (!checkPlaceHolderExist() && this.value == '') {
this.value = this.getAttribute('placeholder');
};
};
};
function checkPlaceHolderExist(obj) {
if (tui.Sys().ie == '6.0' || tui.Sys().ie == '7.0' || tui.Sys().ie == '8.0' || tui.Sys().ie == '9.0') {
return false;
} else {
return true;
}
};
var o = {}
o['cnzz'] = {
'u' : document.getElementById('username_cnzz'),
'p' : document.getElementById('password_cnzz'),
'v' : document.getElementById('verify_cnzz'),
'i' : document.getElementById('verify_input_cnzz'),
'e' : document.getElementById('error_cnzz'),
's' : document.getElementById('submit_cnzz'),
'r' : document.getElementById('login_cnzz_remeber')
};
o['quan'] = {
'c' : document.getElementById('company_quan'),
'u' : document.getElementById('username_quan'),
'p' : document.getElementById('password_quan'),
'v' : document.getElementById('verify_quan'),
'i' : document.getElementById('verify_input_quan'),
'e' : document.getElementById('error_quan'),
's' : document.getElementById('submit_quan'),
'r' : document.getElementById('login_quan_remeber')
};
o['count'] = document.getElementById('login_count');
o['from'] = document.getElementById('from');
o['ajax'] = false;
if (o['count'].value >= 3) {
o['cnzz']['v'].style.visibility = 'visible';
o['quan']['v'].style.visibility = 'visible';
};
o['cnzz']['s'].onclick = function () {
cnzzSubmit();
};
function cnzzSubmit() {
if (o['ajax']) {
tui.Alert('正在请求中,请稍后...');
return;
};
if (o['cnzz']['u'].value == '' || o['cnzz']['u'].value == '请输入用户名') {
o['cnzz']['e'].innerHTML = '请输入用户名';
setTimeout(function () {
o['cnzz']['u'].focus()
}, 0);
} else if (o['cnzz']['p'].value == '' || o['cnzz']['p'].value == '******') {
o['cnzz']['e'].innerHTML = '请输入登陆密码';
setTimeout(function () {
o['cnzz']['p'].focus()
}, 0);
} else if (o['count'].value >= 3 && o['cnzz']['i'].value == '') {
o['cnzz']['e'].innerHTML = '请输入验证码';
setTimeout(function () {
o['cnzz']['i'].focus()
}, 0);
} else {
o['cnzz']['e'].innerHTML = '';
var rem;
o['cnzz']['r'].checked ? rem = 1 : rem = 0;
var pass = o['cnzz']['p'].value;
var pass = pass.replace(/\+/g, "%2B");
var pass = pass.replace(/\&/g, "%26");
$.ajax({
type : 'POST',
url : '?mo=login&fo=login_ok',
data : 'zh_username=' + o['cnzz']['u'].value + '&password=' + pass + '&verify=' + o['cnzz']['i'].value + '&type=1&rem=' + rem + '&from=' + o['from'].value + '&date=' + new Date(),
async : false,
beforeSend : function () {
o['ajax'] = true;
loading.funcShow();
},
success : function (msg) {
o['ajax'] = false;
loading.funcStop();
changepic();
if (msg == 2) {
o['cnzz']['e'].innerHTML = "用户名或密码为空或格式错误";
o['count'].value++;
} else if (msg == 3) {
o['cnzz']['e'].innerHTML = "异常错误";
o['count'].value++;
} else if (msg == 4) {
o['cnzz']['e'].innerHTML = "请输入正确的验证码";
o['count'].value++;
} else if (msg == 1) {
window.location.href = '/intro.html';
} else if (msg == 6) {
o['cnzz']['e'].innerHTML = "用户名或密码错误,请重新输入";
o['count'].value++;
} else if (msg == -8) {
o['cnzz']['e'].innerHTML = "您的登录次数过于频繁,请稍后重试!";
o['count'].value++;
} else if (msg == -9) {
o['cnzz']['e'].innerHTML = "密码错误次数超过10次,请24小时后登录!";
o['count'].value++;
} else if (msg == 7) {
window.location.href = '?mo=rec&fo=rec_style&from=' + o['from'].value;
} else {
window.location.href = '?mo=rec&fo=rec_list';
};
if (o['count'].value >= 3) {
o['cnzz']['v'].style.visibility = 'visible';
o['quan']['v'].style.visibility = 'visible';
};
}
});
};
}
o['quan']['s'].onclick = function () {
quanSubmit();
};
function quanSubmit() {
if (o['ajax']) {
tui.Alert('正在请求中,请稍后...');
return;
};
if (o['quan']['c'].value == '' || o['quan']['c'].value == '请输入公司名') {
o['quan']['e'].innerHTML = '请输入公司名';
setTimeout(function () {
o['quan']['c'].focus()
}, 0);
} else if (o['quan']['u'].value == '' || o['quan']['u'].value == '请输入用户名') {
o['quan']['e'].innerHTML = '请输入用户名';
setTimeout(function () {
o['quan']['u'].focus()
}, 0);
} else if (o['quan']['p'].value == '' || o['quan']['p'].value == '******') {
o['quan']['e'].innerHTML = '请输入登陆密码';
setTimeout(function () {
o['quan']['p'].focus()
}, 0);
} else if (o['count'].value >= 3 && o['quan']['i'].value == '') {
o['quan']['e'].innerHTML = '请输入验证码';
setTimeout(function () {
o['quan']['i'].focus()
}, 0);
} else {
o['quan']['e'].innerHTML = '';
var rem;
o['quan']['r'].checked ? rem = 1 : rem = 0;
var pass = o['quan']['p'].value;
var pass = pass.replace(/\+/g, "%2B");
var pass = pass.replace(/\&/g, "%26");
$.ajax({
type : 'POST',
url : '?mo=login&fo=login_ok',
data : 'qj_username=' + o['quan']['u'].value + '&qj_password=' + pass + '&com_name=' + o['quan']['c'].value + '&verify=' + o['quan']['i'].value + '&type=2&rem=' + rem + '&from=' + o['from'].value + '&date=' + new Date(),
async : false,
beforeSend : function () {
o['ajax'] = true;
loading.funcShow();
},
success : function (msg) {
o['ajax'] = false;
loading.funcStop();
changepic1();
if (msg == 2) {
o['quan']['e'].innerHTML = "公司名或用户名或密码为空或格式错误";
o['count'].value++;
} else if (msg == 3) {
o['quan']['e'].innerHTML = "异常错误";
o['count'].value++;
} else if (msg == 4) {
o['quan']['e'].innerHTML = "请输入正确的验证码";
o['count'].value++;
} else if (msg == 1) {
window.location.href = '/intro.html';
} else if (msg == 6) {
o['quan']['e'].innerHTML = "用户名或密码错误,请重新输入";
o['count'].value++;
} else if (msg == -8) {
o['quan']['e'].innerHTML = "您的登录次数过于频繁,请稍后重试!";
o['count'].value++;
} else if (msg == -9) {
o['quan']['e'].innerHTML = "密码错误次数超过10次,请24小时后登录!";
o['count'].value++;
} else if (msg == 7) {
window.location.href = '?mo=rec&fo=rec_style&from=' + o['from'].value;
} else {
window.location.href = '?mo=rec&fo=rec_list';
};
if (o['count'].value >= 3) {
o['cnzz']['v'].style.visibility = 'visible';
o['quan']['v'].style.visibility = 'visible';
};
}
});
};
};
};
function changepic() {
document.getElementById('validateImg').src = "?mo=login&fo=indentify_code&t=" + Date.parse(new Date());
};
function changepic1() {
document.getElementById('validateImg1').src = "?mo=login&fo=indentify_code&t=" + Date.parse(new Date());
};
function tab() {
var news_m = document.getElementById('news_m').getElementsByTagName('li');
var news_s = document.getElementById('news_s').getElementsByTagName('ul');
news_m[0].onmouseover = function () {
if (this.className != 'li_0') {
this.className = 'active';
news_m[1].className = '';
news_s[0].style.display = 'block';
news_s[1].style.display = 'none';
};
};
news_m[1].onmouseover = function () {
if (this.className != 'li_0') {
this.className = 'active';
news_m[0].className = '';
news_s[1].style.display = 'block';
news_s[0].style.display = 'none';
};
};
};
marqueeTime = null;
var marquee = function () {
var box = document.getElementById('marquee_box');
var o = document.getElementById('marquee').getElementsByTagName('ul')[0];
var li = o.getElementsByTagName('li');
var l = document.getElementById('marquee_l');
var r = document.getElementById('marquee_r');
var m = li.length - 1;
var w = 945;
var aTime = 20;
var sTime = 3000;
var mAuto;
function goPrev() {
clearTimeout(marqueeTime);
startMove(false);
};
function goNext() {
clearTimeout(marqueeTime);
startMove(true);
};
function startMove(type) {
if (type) {
var tt = 0;
} else {
var tt = w;
var t1 = document.createDocumentFragment();
var liArray = [];
for (var i = 6; i >= 0; i--) {
var li = document.createElement('li');
var liTmp = o.getElementsByTagName('li')[m - i];
liArray.push(liTmp)
li.innerHTML = liTmp.innerHTML
t1.appendChild(li);
};
o.insertBefore(t1, o.firstChild);
o.style.marginLeft = '-' + tt + 'px';
for (var i = 0; i < liArray.length; i++) {
o.removeChild(liArray[i]);
};
};
function set() {
if (type) {
if (tt >= w) {
clearInterval(marqueeTime);
var t1 = document.createDocumentFragment();
var liArray = [];
for (var i = 0; i < 7; i++) {
var li = document.createElement('li');
var liTmp = o.getElementsByTagName('li')[i];
liArray.push(liTmp)
li.innerHTML = liTmp.innerHTML
t1.appendChild(li);
};
o.appendChild(t1);
for (var i = 0; i < liArray.length; i++) {
o.removeChild(liArray[i]);
};
o.style.marginLeft = 0;
} else {
o.style.marginLeft = '-' + tt + 'px';
tt += Math.ceil((w - tt) * 0.1);
};
} else {
if (tt <= 0) {
clearInterval(marqueeTime);
o.style.marginLeft = 0;
} else {
o.style.marginLeft = '-' + tt + 'px';
tt = Math.floor(0.9 * tt);
};
}
};
marqueeTime = setInterval(set, aTime);
};
if (li.length < 7) {
l.style.display = r.style.display = 'none'
} else {
r.onclick = function () {
goNext();
};
l.onclick = function () {
goPrev();
};
};
mAuto = setInterval(goNext,sTime);
box.onmouseover = function(){
clearInterval(mAuto);
};
box.onmouseout = function(){
mAuto = setInterval(goNext,sTime);
};
};
var aniBorder = function (){
var o = document.getElementById('tui_header_nav');
var li = o.getElementsByTagName('li');;
var b = document.getElementById('tui_nav_border');
var t;
var n = 0;
var f = 0;
for (var i=0;i<li.length;i++) {
li[i].onmouseover = function (){
mouseGo(tui.getElemPos(this).x);
};
if (li[i].className == 'active') {
n = i;
};
};
o.onmouseout = function (){
mouseGo(tui.getElemPos(li[n]).x);
};
mouseGo(tui.getElemPos(li[n]).x);
function mouseGo(arg) {
arg = arg + 10;
clearInterval(t);
var l = parseInt(b.style.left) || 0;
var ll = l;
t = setInterval(function(){
arg > l ? ll += Math.ceil((arg - ll) * 0.1) : ll += Math.floor((arg - ll) * 0.1)
if (arg > l && ll >= arg || arg <= l && ll <= arg) {
clearInterval(t);
if (!f) {
b.style.display = 'block';
f = 1;
};
}else {
b.style.left = ll + 'px';
}
},10)
};
};
window.onload = function () {
focusRun.init();
marquee();
tab();
aniBorder();
var session = document.getElementById('session');
if (session.value != 'session') {
if (document.getElementById('login_sub')) {
login();
};
};
};
view code
<!--focus start-->
<div class="focus" id="focus">
<div class="login_box">
<div class="login">
<div class="login_bg"></div>
<div class="login_content">
<!--login main start-->
<ul id="login_main" class="login_main">
<li class="li_0"><a title="站长统计用户" hidefocus="true" href="javascript:void(0)" class="active" >站长统计用户</a></li>
<li class="li_1"><a title="全景统计用户" hidefocus="true" href="javascript:void(0)" >全景统计用户</a></li>
</ul>
<!--login main end-->
<!--login sub start-->
<div id="login_sub" class="login_sub">
<!--login_cnzz start-->
<div id="login_cnzz" class="login_cnzz">
<table>
<tbody>
<tr>
<td class="td_0">用户名:</td><td class="td_1"><input type="text" autocomplete="off" name="username_cnzz" id="username_cnzz" value="" tabindex="1" placeholder="请输入用户名"></td>
</tr>
<tr>
<td class="td_0">密 码:</td><td class="td_1"><input type="password" autocomplete="off" name="password_cnzz" id="password_cnzz" value="" tabindex="2" placeholder="******"></td>
</tr>
<tr>
<td class="td_0"></td><td class="td_2"><input type="checkbox" id="login_cnzz_remeber" autocomplete="off"> <label for="login_cnzz_remeber">记住用户名密码</label></td>
</tr>
<tr class="vh" id="verify_cnzz">
<td class="td_0">验证码:</td><td class="td_3"><input type="text" autocomplete="off" name="verify_input_cnzz" id="verify_input_cnzz" maxlength="4"><img height="24" align="absmiddle" width="80" onclick="changepic()" id="validateImg" title="看不清点击更新" alt="看不清点击更新" src="" style="margin-bottom:1px;cursor:pointer;margin-left:10px;"></td>
</tr>
<tr>
<td></td><td class="td_4"><p class="clearFix"><span id="submit_cnzz">登陆</span><a hidefocus="true" class="a_1" title="忘记密码?" href="http://new.cnzz.com/user/get_pass.php">忘记密码?</a></p></td>
</tr>
<tr>
<td colspan="2" id="error_cnzz" class="login_error"></td>
</tr>
</tbody>
</table>
<a class="register" id="register_cnzz" title="注册" hidefocus="true" href="http://new.cnzz.com/user/reg.php?refer=http%3A%2F%2Fzjtui.cnzz.com/?mo=login_ok">注册</a>
</div>
<!--login_cnzz end-->
<!--login_quan start-->
<div id="login_quan" class="login_cnzz none">
<table>
<tbody>
<tr>
<td class="td_0">公司名:</td><td class="td_1"><input type="text" autocomplete="off" name="company_quan" id="company_quan" value="" placeholder="请输入公司名"></td>
</tr>
<tr>
<td class="td_0">用户名:</td><td class="td_1"><input type="text" autocomplete="off" name="username_quan" id="username_quan" value="" placeholder="请输入用户名"></td>
</tr>
<tr>
<td class="td_0">密 码:</td><td class="td_1"><input type="password" autocomplete="off" name="password_quan" id="password_quan" value="" placeholder="******"></td>
</tr>
<tr>
<td class="td_0"></td><td class="td_2"><input type="checkbox" id="login_quan_remeber" autocomplete="off"> <label for="login_quan_remeber">记住公司名、用户名和密码</label></td>
</tr>
<tr class="vh" id="verify_quan">
<td class="td_0">验证码:</td><td class="td_3"><input type="text" autocomplete="off" name="verify_input_quan" id="verify_input_quan" maxlength="4"><img height="24" align="absmiddle" width="80" onclick="changepic1()" id="validateImg1" title="看不清点击更新" alt="看不清点击更新" src="" style="margin-bottom:1px;cursor:pointer;margin-left:10px;"></td>
</tr>
<tr>
<td></td><td class="td_4"><p class="clearFix"><span id="submit_quan">登陆</span></p></td>
</tr>
<tr>
<td colspan="2" id="error_quan" class="login_error"></td>
</tr>
</tbody>
</table>
</div>
<!--login_quan end-->
</div>
<!--login sub end-->
</div>
</div>
</div>
<div id="focus_m" class="focus_m">
<ul>
<li class="li_1"><a href="#" hidefocus="true"></a></li>
<li class="li_2"><a href="#" hidefocus="true"></a></li>
<li class="li_3"><a href="#" hidefocus="true"></a></li>
<li class="li_4"><p><a href="/plugin.html" hidefocus="true" target="_blank" title="插件中心">插件中心</a></p></li>
</ul>
</div>
<a href="javascript:;" class="focus_l" id="focus_l" hidefocus="true" title="上一张"><b></b><span></span></a>
<a href="javascript:;" class="focus_r" id="focus_r" hidefocus="true" title="下一张"><b></b><span></span></a>
</div>
<!--focus end-->
//全局
window.tui = {};
//loading
tui.Loading = new Function();
tui.Loading.prototype = {
init : function (o) {
this.o = o;
this.oImg = document.createElement('div');
this.oImg.className = 'loading';
this.oImg.style.display = 'none';
this.oB = document.createElement('b');
this.oB.innerHTML = 'loading';
this.oImg.appendChild(this.oB);
this.o.appendChild(this.oImg);
if (this.oTop) {
this.oImg.style.top = this.oTop + 'px'
};
},
funcShow : function () {
var that = this;
var i = 0;
this.oImg.style.display = 'block';
this.oAuto = setInterval(loading, 80);
function loading() {
i == 11 ? i = 0 : i++;
that.oB.style.top = "-" + i * 40 + "px"
};
},
funcStop : function () {
clearInterval(this.oAuto);
this.oImg.style.display = 'none';
}
};
//alert
tui.Alert = function (text,title,callBack) {
var o = document.getElementById('alert_mask');
var out = document.getElementById('alert_out');
out.innerHTML = '';
o.style.display = 'none';
title = title || '提示';
out.innerHTML = '<div><h3><b>X</b>' + title + '</h3><h4>' + text + '</h4><p><a href="###" hidefocus="true" title="确定">确定</a></p>';
var a = o.getElementsByTagName('a');
var b = o.getElementsByTagName('b')[0];
o.style.height = Math.max(document.body.offsetHeight, document.body.clientHeight, document.documentElement.clientHeight) + 'px';
out.style.top = (Math.max(document.body.scrollTop, document.documentElement.scrollTop) + 200) + 'px';
b.onclick = a[0].onclick = function () {
out.innerHTML = '';
o.style.display = 'none';
if (callBack) callBack();
};
o.style.display = 'block';
};
//confirm
tui.Confirm = function (set) {
var o = document.getElementById('alert_mask');
var out = document.getElementById('alert_out');
set.title = set.title || '提示';
set.callBack = set.callBack || false;
out.innerHTML = '';
o.style.display = 'none';
out.innerHTML = '<div><h3><b>X</b>' + set.title + '</h3><h4>' + set.text + '</h4><p><a href="###" hidefocus="true" title="确定">确定</a><a href="###" hidefocus="true" title="取消">取消</a></p>';
var a = o.getElementsByTagName('a');
var b = o.getElementsByTagName('b')[0];
o.style.height = Math.max(document.body.offsetHeight, document.body.clientHeight, document.documentElement.clientHeight) + 'px';
out.style.top = (Math.max(document.body.scrollTop, document.documentElement.scrollTop) + 200) + 'px';
b.onclick = a[1].onclick = function () {
out.innerHTML = '';
o.style.display = 'none';
};
a[0].onclick = function () {
out.innerHTML = '';
o.style.display = 'none';
set.callBack();
};
o.style.display = 'block';
};
//addEvent
tui.addEvent = function (Elem, type, handle) {
if (Elem.addEventListener) {
Elem.addEventListener(type, handle, false);
} else if (Elem.attachEvent) {
Elem.attachEvent("on" + type, handle);
};
};
//delEvent
tui.delEvent = function (Elem, type, handle) {
if (Elem.removeEventListener) {
Elem.removeEventListener(type, handle, false);
} else if (Elem.detachEvent) {
Elem.detachEvent("on" + type, handle);
};
};
tui.getElemPos = function (obj) {
var pos = {
"top" : 0,
"left" : 0
};
if (obj.offsetParent) {
while (obj.offsetParent) {
pos.top += obj.offsetTop;
pos.left += obj.offsetLeft;
obj = obj.offsetParent;
}
} else if (obj.x) {
pos.left += obj.x;
} else if (obj.x) {
pos.top += obj.y;
}
return {
x : pos.left,
y : pos.top
};
};
//placeHolder
tui.placeHolder = function (input) {
for (var i = 0; i < input.length; i++) {
input[i].onfocus = function () {
var that = this;
that.className = 'i_0';
setTimeout(function () {
that.select()
}, 0);
};
input[i].onblur = function () {
this.className = '';
};
};
};
//useragent
tui.Sys = function () {
var Sys = {};
var ua = navigator.userAgent.toLowerCase();
var s;
(s = ua.match(/msie ([\d.]+)/)) ? Sys.ie = s[1] :
(s = ua.match(/firefox\/([\d.]+)/)) ? Sys.firefox = s[1] :
(s = ua.match(/chrome\/([\d.]+)/)) ? Sys.chrome = s[1] :
(s = ua.match(/opera.([\d.]+)/)) ? Sys.opera = s[1] :
(s = ua.match(/version\/([\d.]+).*safari/)) ? Sys.safari = s[1] : 0;
return Sys;
};
//正则
tui.regular = function (type, value) {
//正则表达式
var regular = {};
//域名
//regular['domain']=/^([a-zA-Z0-9]([a-zA-Z0-9\-]{0,61}[a-zA-Z0-9])?\.)+[a-zA-Z]{2,6}$/;
var strRegex = "^((https|http|ftp|rtsp|mms)?://)"
+ "?(([0-9a-zA-Z_!~*'().&=+$%-]+: )?[0-9a-z_!~*'().&=+$%-]+@)?" //ftp的user@
+ "(([0-9]{1,3}\.){3}[0-9]{1,3}" // IP形式的URL- 199.194.52.184
+ "|" // 允许IP和DOMAIN(域名)
+ "([0-9a-zA-Z_!~*'()-]+\.)*" // 域名- www.
+ "([0-9a-zA-Z][0-9a-z-]{0,61})?[0-9a-z]\." // 二级域名
+ "[a-zA-Z]{2,6})" // first level domain- .com or .museum
+ "(:[0-9]{1,4})?" // 端口- :80
+ "((/?)|" // a slash isn't required if there is no file name
+ "(/[0-9a-zA-Z_!~*'().;?:@&=+$,%#-]+)+/?)$";
regular['domain'] = new RegExp(strRegex);
//二级域名
regular['subDomain'] = /([0-9a-zA-Z][0-9a-z-]{0,61})?[0-9a-zA-Z]\./;
//正整数
regular['num'] = /^[1-9]\d*$/;
//中文 英文 数字
regular['text'] = /^([\u4E00-\uFA29]|[\uE7C7-\uE7F3]|[\w])*$/;
regular['puretxt'] = /^[\u4e00-\u9fa5 a-zA-Z0-9]+$/;
//邮箱
regular['email'] = /^([a-zA-Z0-9_-])+@([a-zA-Z0-9_-])+((\.[a-zA-Z0-9_-]{2,3}){1,2})$/;
regular['txt'] = /^[\u4e00-\u9fa5 ,\.;\:\"\'a-zA-Z0-9]+$/;
regular['truDomain'] = new RegExp(/^((https|http|ftp|rtsp|mms)?:\/\/)?([a-zA-Z0-9]([a-zA-Z0-9\-]{0,61}[a-zA-Z0-9])?\.)+[a-zA-Z]{2,6}$/);
return regular[type].test(value);
};
function Sys() {
var Sys = {};
var ua = navigator.userAgent.toLowerCase();
var s;
(s = ua.match(/msie ([\d.]+)/)) ? Sys.ie = s[1] :
(s = ua.match(/firefox\/([\d.]+)/)) ? Sys.firefox = s[1] :
(s = ua.match(/chrome\/([\d.]+)/)) ? Sys.chrome = s[1] :
(s = ua.match(/opera.([\d.]+)/)) ? Sys.opera = s[1] :
(s = ua.match(/version\/([\d.]+).*safari/)) ? Sys.safari = s[1] : 0;
return Sys;
};
function addEvent(Elem, type, handle) {
if (Elem.addEventListener) {
Elem.addEventListener(type, handle, false);
} else if (Elem.attachEvent) {
Elem.attachEvent("on" + type, handle);
};
};
tui.help_pop = function (node) {
if (node) {
var b = node.getElementsByTagName('b');
var h = document.getElementById('help_box');
var a = document.getElementById('help_arrow');
var p = h.getElementsByTagName('div')[0];
for (var i = 0; i < b.length; i++) {
if (!b[i].getAttribute('jsdata') || b[i].getAttribute('jsdata') =='') continue;
b[i].onmouseover = function () {
var txt = this.getAttribute('jsdata');
this.className = 'active';
p.innerHTML = txt;
var l = tui.getElemPos(this).x - 20;
var ll = (l + 312 - Math.max(document.body.clientWidth, document.documentElement.clientWidth));
if (ll > 0) {
h.style.left = (l - ll) + 'px';
a.style.left = (22 + ll) + 'px';
} else {
h.style.left = l + 'px';
a.style.left = '22px';
};
h.style.display = 'block';
var t = tui.getElemPos(this).y - parseInt(p.offsetHeight + 10);
h.style.top = t + 'px';
};
b[i].onmouseout = function () {
this.className = '';
p.innerHTML = '';
h.style.display = 'none';
};
};
};
}
view code
关注我
我的微信公众号:前端开发博客,在后台回复以下关键字可以获取资源。
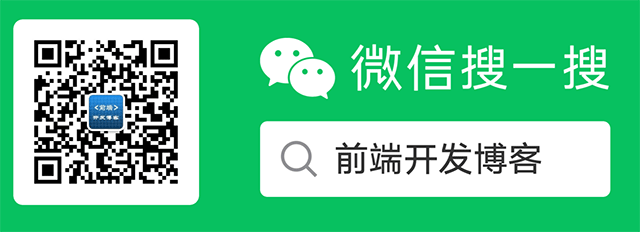
-
回复「小抄」,领取Vue、JavaScript 和 WebComponent 小抄 PDF
-
回复「Vue脑图」获取 Vue 相关脑图
-
回复「思维图」获取 JavaScript 相关思维图
-
回复「简历」获取简历制作建议
-
回复「简历模板」获取精选的简历模板
-
回复「加群」进入500人前端精英群
-
回复「电子书」下载我整理的大量前端资源,含面试、Vue实战项目、CSS和JavaScript电子书等。
-
回复「知识点」下载高清JavaScript知识点图谱
每日分享有用的前端开发知识,加我微信:caibaojian89 交流